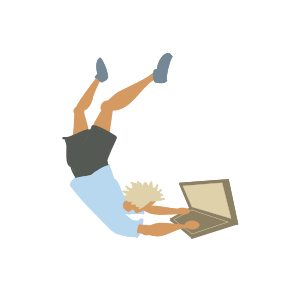
Diff Your Changes
We have reviewed how to push changes, and how to delete files in a repository (which essentially is the same).
In this article you will learn “to diff your work”. git diff
is a command to compare differences between two files, which comes in very handy to review what changes you’ve done. It is very useful to avoid committing changes that are not desired.
We all write portions of code for testing purposes and when writing them we think “I will delete this before adding the changes”. If we forget to remove them and push the changes, even when they don’t affect the program as a whole, they affect our repository history. Yes, you can always remove the undesired changes in the next commit, but with this approach, it will be harder in the future for you or another programmer to understand the history of the project - that’s why you should always diff your work before adding the changes.
We’ll see how git diff
works with a very simple example. Let’s start by reviewing the files in the repository, and the status.
$ git status
On branch main
Your branch is up to date with 'origin/main'.
nothing to commit, working tree clean
At the moment there are no changes to commit, and the files in the repository are:
$ ls
newfile.txt README.md
Just two files. Let’s start by modifying one of them. If I look at the content of newfile.txt
:
$ cat newfile.txt
hello world
It only has a line with the text “hello world”. I will add a new line:
$ echo "this is a new text" >> newfile.txt
$ cat newfile.txt
hello world
this is a new text
We have modified a file in the repository. Let’s check the status again
$ git status
On branch main
Your branch is up to date with 'origin/main'.
Changes not staged for commit:
(use "git add <file>..." to update what will be committed)
(use "git restore <file>..." to discard changes in working directory)
modified: newfile.txt
no changes added to commit (use "git add" and/or "git commit -a")
There it is. The change is not staged for commit but git status
identifies it. If we use git diff
, we will be able to see exactly what changed:
$ git diff
diff --git a/newfile.txt b/newfile.txt
index 3b18e51..64fa5d7 100644
--- a/newfile.txt
+++ b/newfile.txt
@@ -1 +1,2 @@
hello world
+this is a new text
The first time you read the output of git diff
it can look confusing, but you will get used to it very quickly. Look at the +
symbol before this is a new text
. The +
means that a new line was added. Let’s now delete a line from the file and see how the git diff
output changes.
$ sed -i '1d' newfile.txt
$ cat newfile.txt
this is a new text
$ git diff
diff --git a/newfile.txt b/newfile.txt
index 3b18e51..32a51e6 100644
--- a/newfile.txt
+++ b/newfile.txt
@@ -1 +1 @@
-hello world
+this is a new text
The new output of the git diff
has a -
before hello world
, because we deleted that line from the file. I will now commit and push the changes, so you can also see them in the GitHub web app.
$ git add .
$ git commit -m "add changes from diff article"
[main e4d6c98] add changes from diff article
1 file changed, 1 insertion(+), 1 deletion(-)
$ git push origin main
Enumerating objects: 5, done.
Counting objects: 100% (5/5), done.
Delta compression using up to 12 threads
Compressing objects: 100% (2/2), done.
Writing objects: 100% (3/3), 300 bytes | 300.00 KiB/s, done.
Total 3 (delta 0), reused 0 (delta 0)
To https://github.com/lmponcio/aits-git.git
7e4a4e7..e4d6c98 main -> main
If you go to the commit in the GitHub web app you will see the changes containing -
and +
before each line that was removed or added.
git diff
has many options that are not covered in this article. Please refer to the documentation to learn more.